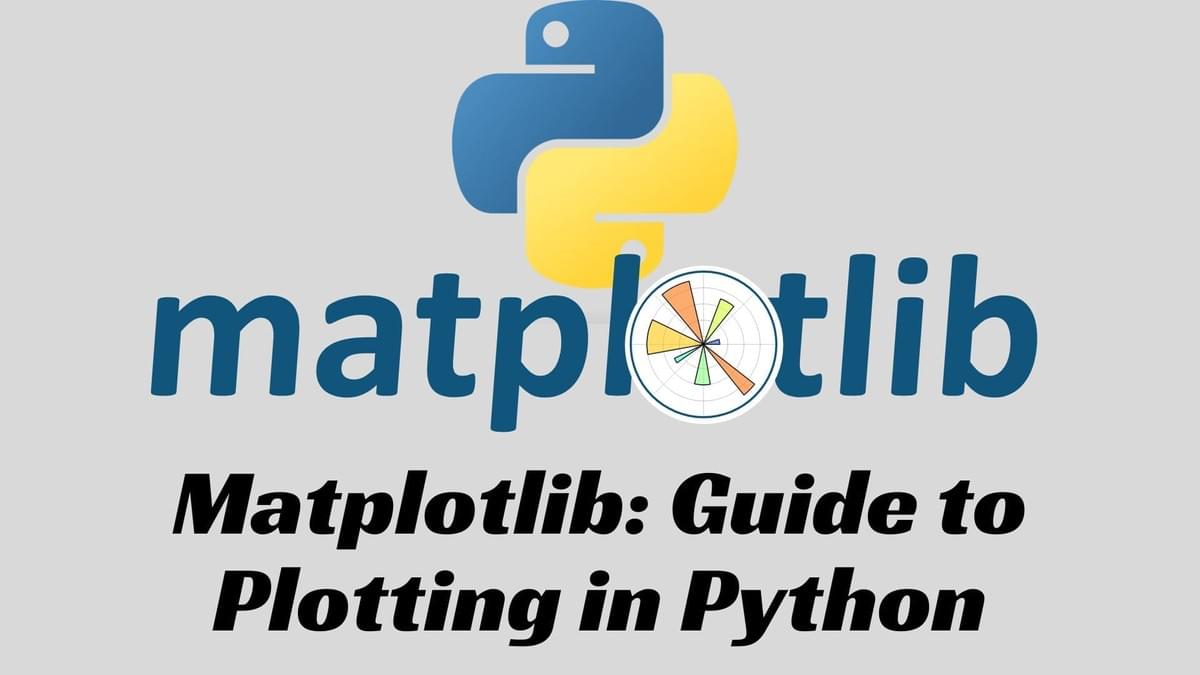
Matplotlib stands as one of the most widely used visualization libraries in Python. Whether you're a data scientist, a researcher, or a student, chances are you'll need to create visualizations at some point. Matplotlib provides a powerful yet straightforward way to generate plots, graphs, and other visual representations of data.
What Matplotlib is, its key features, and how to leverage it effectively for plotting data in Python.
What is Matplotlib?
Matplotlib is a versatile plotting library for Python thatwas created by John D. Hunter. It provides a MATLAB-like interface for creating static, animated, and interactive visualizations in Python. Matplotlib is open-source and is widely used in various fields such as data science, machine
learning, scientific computing, and more.
You can also read: Data analyst course in Bangalore
### Key Features of Matplotlib:
1. **Simple Interface:** Matplotlib provides a simple andintuitive interface for creating a wide range of plots with just a few lines of code.
2. **Customization:** Users have full control over everyaspect of the plot, including line styles, colors, markers, labels, annotations, and more.
3. **Compatibility:** Matplotlib seamlessly integrates withother Python libraries such as NumPy, Pandas, and SciPy, making it easy to visualize data stored in these formats.
4. **Publication-Quality Plots:** Matplotlib is capable ofproducing high-quality plots suitable for publication in academic journals, reports, and presentations.
5. **Wide Range of Plot Types:** Matplotlib supports variousplot types, including line plots, scatter plots, bar plots, histograms, pie charts, 3D plots, and more.
### How to Install Matplotlib:
Before using Matplotlib, you need to install it. You caninstall Matplotlib using pip, the Python package installer, by executing the following command in your terminal or command prompt:
```bash
pip install matplotlib
```
You can also read: Data science course in Chennai
### Getting Started with Matplotlib:
To start using Matplotlib for plotting, you first need toimport the library. The convention is to import it under the alias `plt`:
```python
import matplotlib.pyplot as plt
```
Once imported, you can begin creating plots. Let's gothrough some common types of plots and how to create them using Matplotlib:
1. **Line Plot:**
A line plot is useful for visualizing trends over time orany continuous data.
```python
import matplotlib.pyplot as plt
You can also read: Data science course in India
# Data
x = [1, 2, 3, 4, 5]
y = [2, 4, 6, 8, 10]
# Create a line plot
plt.plot(x, y)
# Add labels and title
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.title('Line Plot Example')
# Show plot
plt.show()
```
You can also read: Data science course in Delhi
2. **Scatter Plot:**
A scatter plot is used to visualize the relationship betweentwo variables.
```python
import matplotlib.pyplot as plt
# Data
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
# Create a scatter plot
plt.scatter(x, y)
# Add labels and title
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.title('Scatter Plot Example')
# Show plot
plt.show()
```
You can also read: Best Online Data ScienceCourse with Training
3. **Bar Plot:**
A bar plot is useful for comparing categories of data.
```python
import matplotlib.pyplot as plt
# Data
x = ['A', 'B', 'C', 'D']
y = [10, 20, 15, 25]
# Create a bar plot
plt.bar(x, y)
# Add labels and title
plt.xlabel('Categories')
plt.ylabel('Values')
plt.title('Bar Plot Example')
# Show plot
plt.show()
```
4. **Histogram:**
A histogram is used to visualize the distribution of acontinuous variable.
```python
import matplotlib.pyplot as plt
import numpy as np
# Generate random data
data = np.random.randn(1000)
# Create a histogram
plt.hist(data, bins=30, edgecolor='black')
# Add labels and title
plt.xlabel('Values')
plt.ylabel('Frequency')
plt.title('Histogram Example')
# Show plot
plt.show()
```
You can also read: Best Data Science CoursesOnline
### Conclusion:
Matplotlib is an indispensable tool for datavisualization in Python. Its simplicity, flexibility, and extensive documentation make it the go-to library for creating a wide range of plots. In this guide, we've covered the basics of Matplotlib, including its features, installation process, and how to create various types of plots.